What Does "Exploring the Power of Python's URL Parser for Web Scraping" Do?
URL Parsing Produced Easy along with Python: Tips and Secrets
Parsing URLs may be a daunting task, especially when dealing along with lengthy and complicated URLs. Thankfully, Python provides many built-in collections that simplify this procedure. In this post, we will discover how to parse URLs utilizing Python.
URL Parsing
Parsing a URL suggests dividing its a variety of parts right into their respective parts. These components include the system, hostname, port amount (if any), pathway, inquiry specifications, and piece identifier.
Python has actually built-in public libraries that give functionality to analyze URLs in different techniques. Answers Shown Here of these collections feature:
1. urllib.parse
2. urlparse
3. urlsplit
urllib.parse Library
The urllib.parse public library in Python delivers the urlparse() feature which can be used to analyze a URL right into its elements.
To utilize this functionality, we initially need to import it through working the observing code:
```python
from urllib.parse import urlparse
```
Once we have imported this functionality, we can easily analyze a URL through phoning it with the URL as an debate:
```python
url = "https://www.example.com/road/to/page?param1=value1¶m2=value2#fragment"
parsed_url = urlparse(url)
```
After phoning urlparse(), the parsed_url object will definitely contain all of the various components of the URL.
We can access each element utilizing qualities on the parsed_url object as revealed below:
```python
system = parsed_url.scheme # 'https'
hostname = parsed_url.hostname # 'www.example.com'
road = parsed_url.path # '/path/to/page'
query_params = parsed_url.query # 'param1=value1¶m2=value2'
fragment_identifier = parsed_url.fragment # 'particle'
```
urlparse Library
The urlparse library is yet another built-in public library in Python that offers similar capability for parsing URLs.
To make use of this collection and its functionality, we first need to have to import it through running the observing code:
```python
from urlparse import urlparse
```
Once we have imported this library, we may analyze a URL through getting in touch with the urlparse() functionality along with the URL as an debate:
```python
url = "https://www.example.com/road/to/page?param1=value1¶m2=value2#fragment"
parsed_url = urlparse(url)
```
After contacting urlparse(), the parsed_url item are going to contain all of the different elements of the URL.
We can access each component utilizing qualities on the parsed_url item as presented listed below:
```python
scheme = parsed_url.scheme # 'https'
hostname = parsed_url.hostname # 'www.example.com'
course = parsed_url.path # '/path/to/page'
query_params = parsed_url.query # 'param1=value1¶m2=value2'
fragment_identifier = parsed_url.fragment # 'particle'
```
urlsplit Library
The urlsplit collection is another built-in collection in Python that supplies comparable performance for parsing URLs.
To make use of this library and its functionality, we to begin with need to import it through functioning the adhering to code:
```python
coming from urllib.parse import urlsplit
```
Once we have imported this public library, we can parse a URL by getting in touch with the urlsplit() functionality with the URL as an argument:
```python
url = "https://www.example.com/pathway/to/page?param1=value1¶m2=value2#fragment"
parsed_url = urlsplit(url)
```
After getting in touch with urlsplit(), the parsed_url object will consist of all of the various parts of the URL.
We may access each element making use of characteristics on the parsed_url item as shown below:
```python
system = parsed_url.scheme # 'https'
hostname = parsed_url.hostname # 'www.example.com'
road = parsed_url.path # '/path/to/page'
query_params = parsed_url.query # 'param1=value1¶m2=value2'
fragment_identifier = parsed_url.fragment # 'particle'
```
Parsing Query Criteria
Question guidelines are the name-value sets that adhere to the inquiry mark in a URL. They are utilized to pass record to the server via HTTP GET asks for.
To parse query guidelines using Python, we can make use of the urllib.parse library. Exclusively, we can easily make use of the parse_qs() feature to parse inquiry criteria right into a thesaurus.
```python
coming from urllib.parse import urlparse, parse_qs
url = "https://www.example.com/road/to/page?param1=value1¶m2=value2#fragment"
parsed_url = urlparse(url)
query_params = parsed_url.query
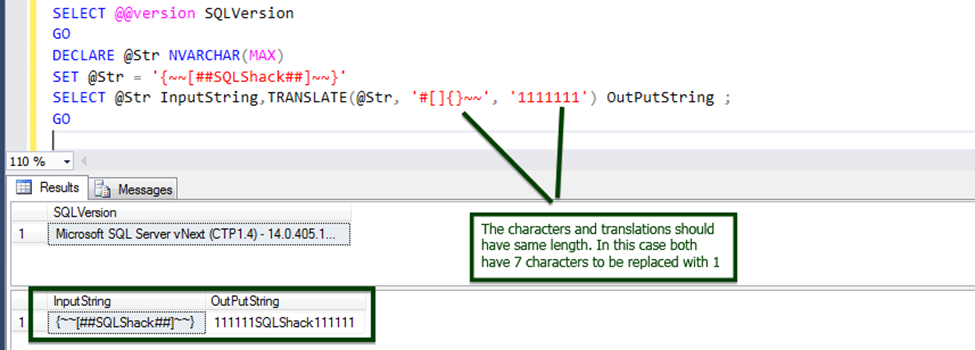
parsed_query_params = parse_qs(query_params)
# Printing out each specification and its worth
for param in parsed_query_params:
print(param+":" + str(parsed_query_params[param]))
```
The output of this code will be:
```
param1: ['value1']
param2: ['value2']
```
Verdict
In final thought, parsing URLs is an essential job when working along with internet applications. Python provides numerous built-in public libraries that streamline this process. Through making use of these public libraries, you can easily effortlessly extract various parts of a URL and operate with them in your code.